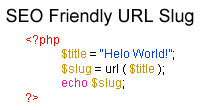
Creating SEO-Friendly URL from Title (WordPress like URL Slug) in PHP
The URL Slug is a converted version of a Post or an article title, comprised of small letters, numbers, and hyphens only. There are many techniques for converting the title string into the slug, and often webmasters type the slug manually in their projects. The main problem for them is refining the title to automatically convert into URL eliminating the need to manually writing one.
That SEO Friendly URL Slug, and in a simpler way, it can be generated via the following steps:
Lets start by defining a title/string
$title = " Hello! This is Tutzi & you're reading a URL_Slug php-tutorial. ";
Step 1: Convert the Title/String to Lower-case
$url = strtolower($title); // Output: hello! this is tutzi & you're reading a url_slug php-tutorial.
Step 2: Convert Unwanted Characters Spaces
We will now work on $url since the content of $title has been copied $url in lower case.
All characters except 0-9 and a-z need to be converted into spaces
$url = preg_replace("/[^a-z0-9]/i", " ", $url); // Output: hello this is tutzi you re reading a url slug php tutorial
Step 3: Remove Multiple Whitespaces
$url = preg_replace('/\s\s+/', ' ', $url); // Output: hello this is tutzi you re reading a url slug php tutorial
Step 4: Remove Whitespaces From the Beginning and the End of Url
//From Beginning if($url{0} == " ") { $url = substr($url, 1); } //From End if(substr($url, -1) == " ") { $url = substr($url,0,strlen($url)-1); } //Output: hello this is tutzi you re reading a url slug php tutorial
Step 5: Convert Whitespaces to Dashes
$url = str_replace(" ","-",$url); // Output: hello-this-is-tutzi-you-re-reading-a-url-slug-php-tutorial
The Final Function and Usage
function url($title) { $url = strtolower($title); $url = preg_replace("/[^a-z0-9]/i", " ", $url); $url = preg_replace('/\s\s+/', ' ', $url); if($url{0} == " ") { $url = substr($url, 1); } if(substr($url, -1) == " ") { $url = substr($url,0,strlen($url)-1); } $url = str_replace(" ","-",$url); return $url; } // Usage $title = " Hello! This is Tutzi ^ you're reading a URL_Slug php-tutorial. "; $slug = url($title); echo $slug;